
Domains
Get your name on the Internet!
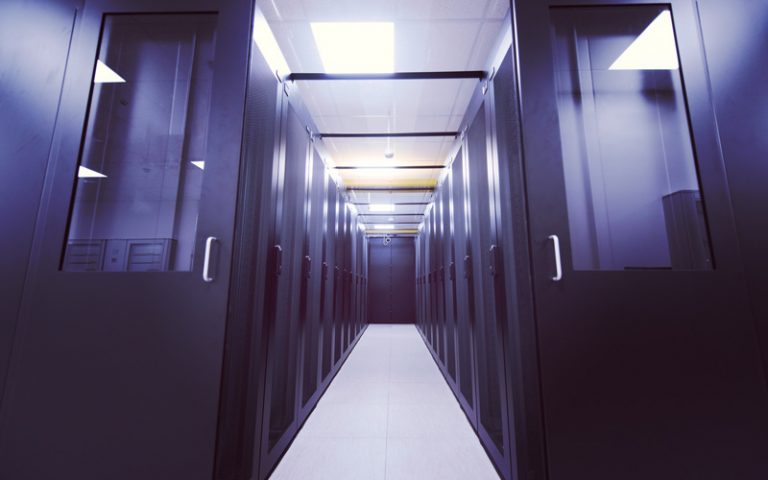
Hosting Features
From the business owner to the individual who desires full functionality on a small budget, Servage provides your complete web hosting solution.

Contact
What can we do for you?

About Servage
Thank you for taking this opportunity to learn more about your friends at Servage. We are always proud when current or potential customers take time to read more about our company